In this article we’ll look at how to create a list of objects and display them in React. I won’t take you through the preamble of setting up your React environment. I’ll just focus on creating the list and displaying it.
Creating the List of Objects
In this example, we will create a simple list of books. Each book will have a title, author, and year of publication. For the sake of simplicity, we’ll hardcode the list of books in our React component. In a real-world application, this data would likely come from an API or database.
Open the src
folder and create a new file named BookList.js
. In this file, define an array of book objects:
// src/BookList.js
const books = [
{
title: "Clean Code: A Handbook of Agile Software Craftsmanship",
author: "Robert C. Martin",
year: 2008,
},
{
title: "Design Patterns: Elements of Reusable Object-Oriented Software",
author: "Erich Gamma, Richard Helm, Ralph Johnson, John Vlissides",
year: 1994,
},
{
title: "The Pragmatic Programmer: Your Journey to Mastery",
author: "Andrew Hunt, David Thomas",
year: 1999,
},
{
title: "Refactoring: Improving the Design of Existing Code",
author: "Martin Fowler, Kent Beck, John Brant, William Opdyke, Don Roberts, Erich Gamma",
year: 1999,
},
{
title: "Domain-Driven Design: Tackling Complexity in the Heart of Software",
author: "Eric Evans",
year: 2003,
},
// Add more books if desired
];
export default books;
Displaying the List of Objects
Next, let’s create a React component that will display the list of books we defined in the BookList.js
file. Create a new file named BookDisplay.js
in the src
folder:
// src/BookDisplay.js
import books from "./BookList";
const BookDisplay = () => {
return (
<div>
<h1>List of Books</h1>
<ul>
{books.map((book, index) => (
<li key={index}>
<strong>Title:</strong> {book.title}<br />
<strong>Author:</strong> {book.author}<br />
<strong>Year:</strong> {book.year}
</li>
))}
</ul>
</div>
);
};
export default BookDisplay;
In the BookDisplay.js
component, we import the books
array from BookList.js
and use the map
function to iterate over the array. For each book, we render a list item (<li>
) with the book’s title, author, and year of publication.
Integrating the BookDisplay Component
Now that we have our BookDisplay
component ready, we need to integrate it into the main App
component so that it appears on the web page. Open the src/App.js
file and update it as follows:
// src/App.js
import BookDisplay from "./BookDisplay";
const App = () => {
return (
<div className="App">
<BookDisplay />
</div>
);
};
export default App;
Running the Application
With everything set up, it’s time to run the React application. In your terminal or command prompt, make sure you are in the root folder of your project and run:
npm start
This will start the development server, and your web browser should open automatically, displaying the list of books.
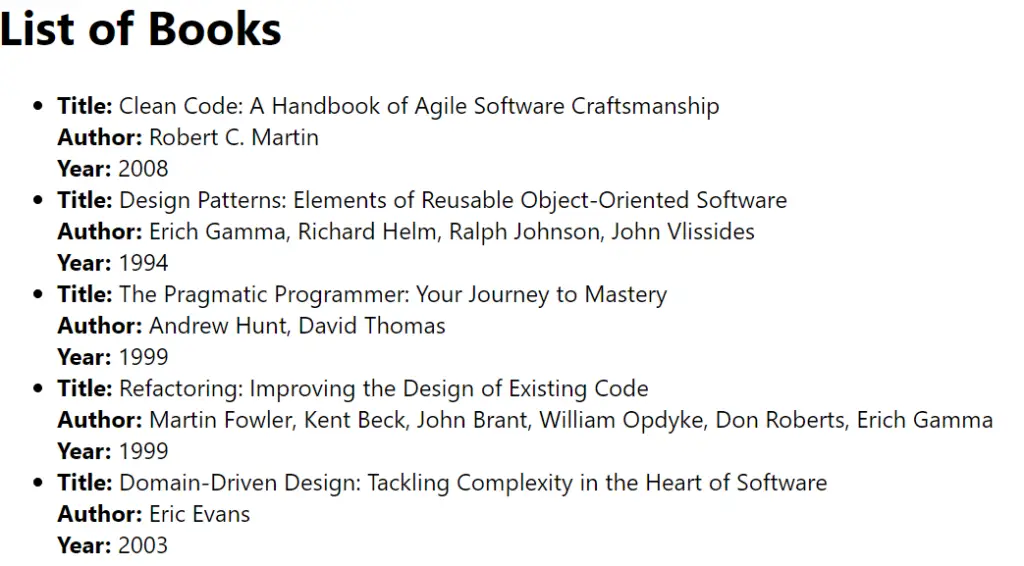
Conclusion
In this article, we covered how to create a list of objects and display them in a React application. We utilized React components, imported and rendered data, and iterated over the list using the map
function. With this basic understanding, you can extend this concept to handle more complex data and build sophisticated user interfaces in your React projects. Happy coding!
Don’t forget to check out my other React articles here or follow me on X/Twitter