Tree shaking is a technique used in computer programming, particularly in the context of modern JavaScript bundlers and compilers, to eliminate unused or “dead” code from the final output bundle or executable.
The goal is to optimize the size and performance of the resulting code by removing portions of the code that are not used in the application, thereby reducing the overall footprint of the software.
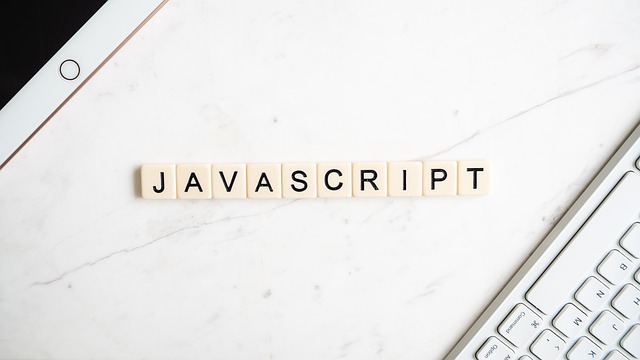
Tree shaking works by analyzing the static code dependencies of an application and identifying portions of the code that are not reachable from the entry point or are not used in any meaningful way. These unused code segments are eliminated during the build process. This results in a smaller output bundle containing only the code necessary for the application to function correctly.
This can result in faster load times, reduced bandwidth usage, and improved runtime performance.
Tree shaking relies on modern JavaScript language features such as ES6 modules, which allow for static analysis of imports and exports, and a build tool or a bundler that supports tree shaking, such as Webpack or Rollup.
Additionally, developers need to write code modular and optimized manner. Using clearly defined imports and exports enables effective tree shaking.
Overall, this is an important optimization technique in modern web development workflows. It helps to reduce the size and improve the performance of JavaScript applications, resulting in a better user experience.
A Tree Shaking Example
Let’s look at a concrete example of this in JavaScript.
If we had a file that contained four string functions:
- concat
- substr
- toUpper
- toLower
export const concat = (a, b) => a.concat(b);
export const substr = (str, start, length) => str.substr(start, length);
export const toUpper = (str) => str.toUpperCase();
export const toLower = (str) => str.toLowerCase();
And we had an application that only used concat and substr:
import { concat, substr } from './math.js';
console.log(concat('Hello', 'World'));
console.log(substr('Hello', 1, 3));
When we bundle app.js
with a tree-shaking-enabled bundler, the unused functions toUpper
and toLower
from the math.js
module will be eliminated from the final bundle, resulting in a smaller and more efficient JavaScript bundle with only the used functions concat
and substr
.
Thanks for reading! Don’t forget to check out our articles on React here or to chase me down on Twitter