In no particular order, here are the ten libraries that I think every React dev should at least know about.
1. MobX
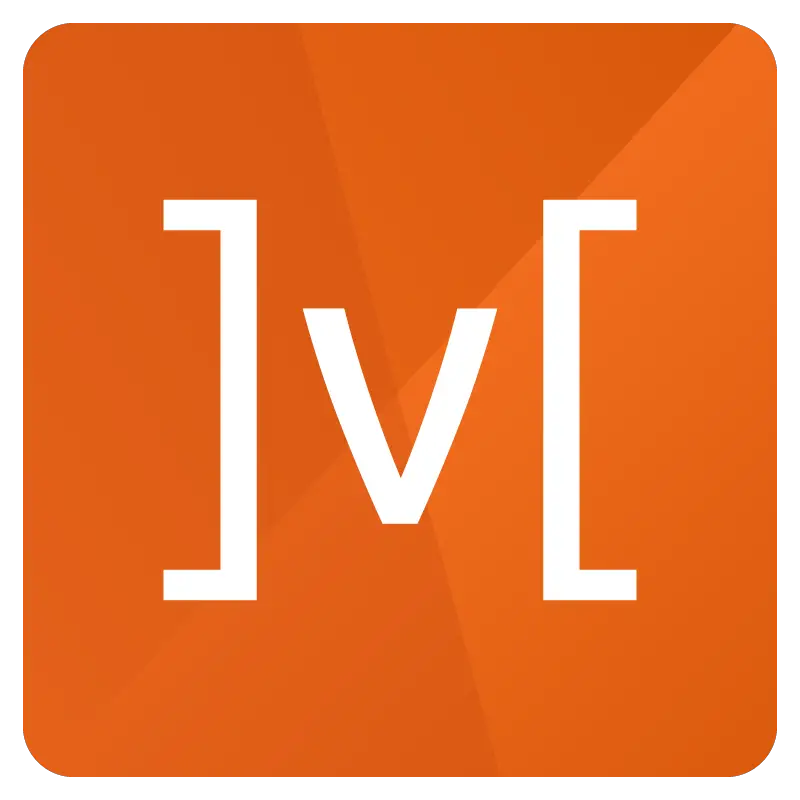
This is a library for managing state in JavaScript applications. It helps you keep track of changes to your data and automatically updates your user interface when those changes occur.
Think of it like a magic assistant sitting in your code and watching everything happening. Whenever something changes, it waves its wand and updates your app accordingly. You don’t have to worry about manually updating the interface or keeping track of all the different pieces of data. MobX takes care of it for you.
This makes it much easier to build complex applications. Because you can focus on writing the code that makes your app work. Rather than worrying about the plumbing that connects everything. With MobX, you can create reactive, responsive apps that feel snappy and fast.Eeven when dealing with large amounts of data.
2. MUI Core
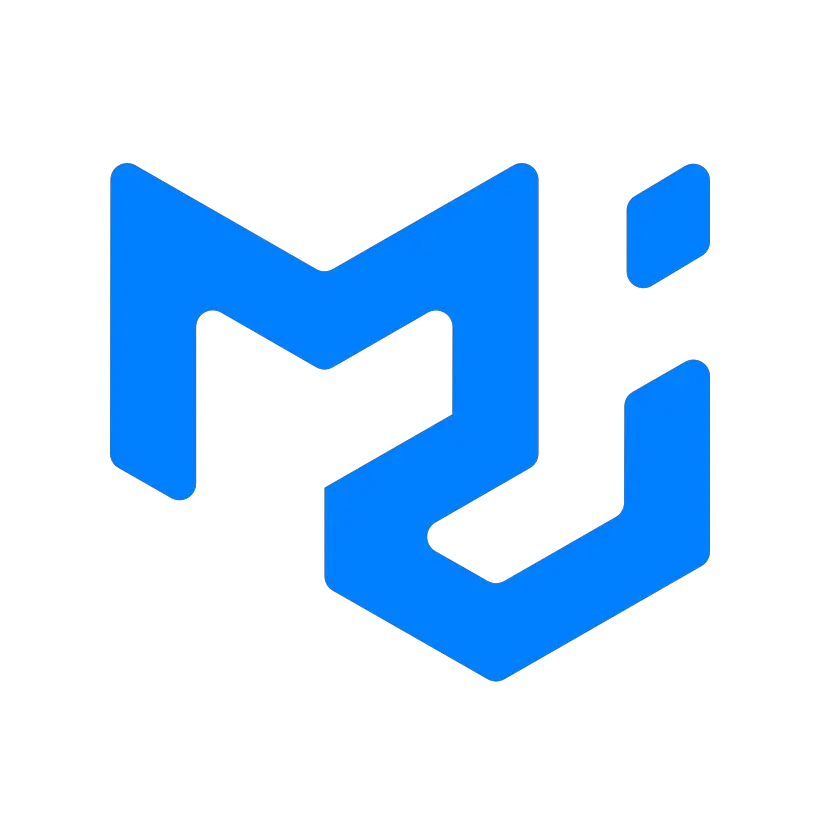
Also known as Material-UI, this is a popular open-source UI component library for React based on Google’s Material Design principles. Providing pre-built and customizable UI components such as buttons, forms, icons, typography, and more. Developers can use these to quickly build modern and visually appealing web applications.
It follows a component-based architecture, allowing developers to easily create reusable and composable UI components. It also provides theming support, allowing developers to customize the look and feel of their applications. This is done by defining their colour palette, typography, and other design elements.
MUI Core is widely used in the React community and has a large and active community of contributors. It is continuously updated and improved, with new features and components regularly added. Licensed under the MIT License, making it free to use and modify in commercial and non-commercial projects.
3. Styled Components
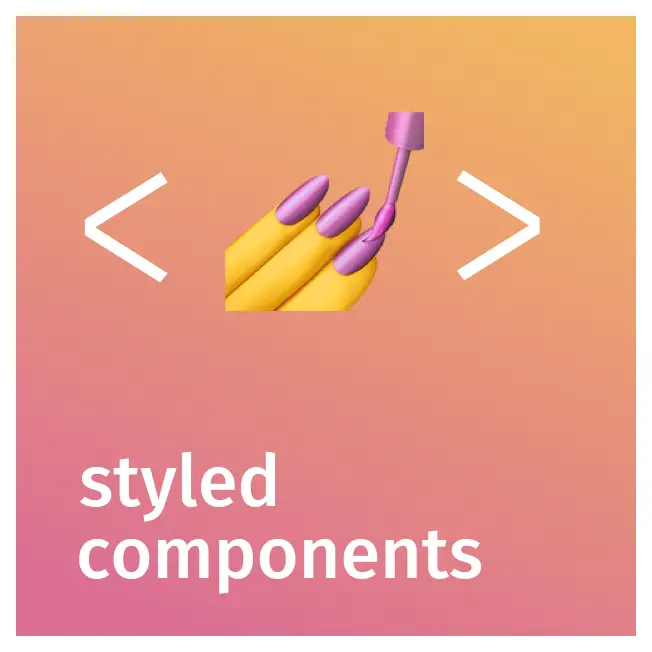
A popular CSS-in-JS library for React that allows developers to write CSS code inside their React components. It provides a way to style components more modularly and reusable way. The styles are encapsulated within the component. Therefore they don’t affect other components of the application.
This library allows developers to define styles as JavaScript functions or tagged template literals. These styles are then applied to the corresponding components using a higher-order component (HOC) or a styled component factory function. This makes it easy to create responsive and dynamic styles based on props or state.
It also supports server-side rendering, theming, and automatic vendor prefixing. Making it a versatile and powerful tool for styling React components.
One of the advantages of using this is that it allows developers to write CSS in a more maintainable way. By keeping styling close to the components they style, it becomes easier to reason about how style changes will affect the component and its children. Additionally, this library allows developers to use all the power of JavaScript to create dynamic and complex styles. This makes it a very flexible and powerful tool for building modern web applications.
Could you think of how styled components could be used in our Card component?
4. react-icons
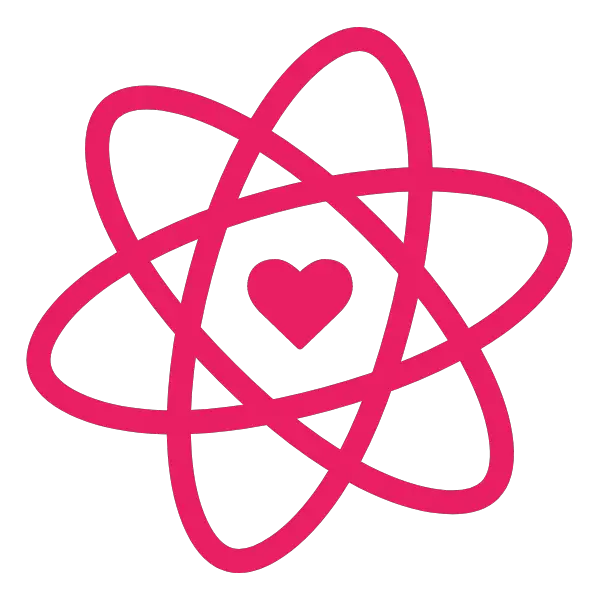
React Icons is a popular open-source library that provides a set of customizable icons for use in React applications. It offers a wide range of icons from popular icon sets such as FontAwesome, Material Design, and Ionicons, as well as custom-designed icons.
React Icons provides a simple and flexible API that allows developers to add icons to their components easily. It supports multiple sizes, colours, and styles for each icon and provides different ways to import and use icons depending on the developer’s preferences.
Some of the advantages of using React Icons include the following:
- Customizable: React Icons provides a simple and flexible way to customize icons by changing their size, colour, and style.
- Easy to use: The library provides a straightforward API that makes adding icons to React components easy.
- Lightweight: The library is lightweight and does not require any additional dependencies.
- Widely used: React Icons is a popular library with a large and active community of contributors. Making it a reliable and well-maintained option for adding icons to React applications.
Summing up, React Icons is a great tool for developers who need to add icons to their React applications in a flexible and customizable way.
5. react-query

React Query is a popular open-source library for managing server-state and fetching data in React applications. It offers a simple and intuitive API for data fetching, caching, and synchronization, making handling complex data requirements in your React components easier.
React Query offers key features that make it a powerful tool for managing data in your React applications. In particular, these include:
- Query caching: React Query automatically caches your API responses. This can improve the performance of your application and reduce the number of network requests.
- Data synchronization: React Query provides built-in support for real-time polling, and optimistic updates, making it easier to keep your application in sync with your backend.
- Error handling: React Query offers robust error handling, built-in support for retrying failed requests, handling stale data, and more.
- Pagination and infinite scrolling: React Query provides built-in support for pagination and infinite scrolling, making it easy to handle large data sets.
Overall, React Query simplifies data fetching and management in React applications, helping developers to build more performant and reliable user interfaces.
6. react-table
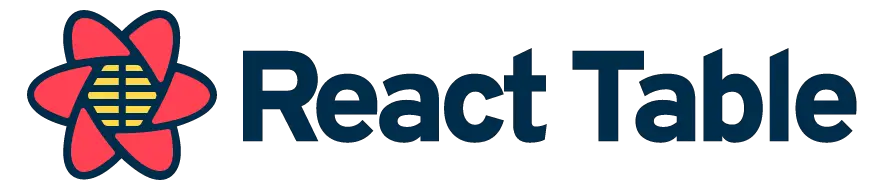
React Table is a popular open-source library for building data tables in React applications. It provides a flexible and customizable API for rendering tables with large amounts of data, allowing developers to create powerful and performant user interfaces.
Some of the key features of React Table include:
- Sorting and filtering: React Table provides built-in support for sorting and filtering data, making it easy to display large data sets meaningfully.
- Pagination: React Table supports pagination, allowing you to display data in smaller, more manageable chunks.
- Row selection: React Table supports row selection, making implementing features like bulk actions and editing easy.
- Custom rendering: React Table provides a powerful API for customizing your table cells, headers, and more rendering.
- Server-side rendering: React Table supports server-side rendering, allowing you to render your tables on the server and send the HTML to the client for improved performance and accessibility.
Evidently, React Table is highly customizable and extensible, making it a popular choice for building data tables in React applications. It is also compatible with various data sources, including JSON, CSV, and REST APIs.
7. create-react-app (CRA)
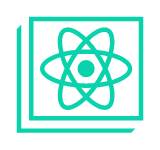
Create React App (CRA) is an open-source tool created by Facebook for setting up a modern React application with zero build configuration. It provides a simple and streamlined way to create and manage a new React project, allowing developers to focus on building their applications instead of worrying about tooling and configuration.
Create React App sets up a development environment for React that includes many of the commonly-used tools and technologies, such as:
- Webpack: A module bundler that helps to bundle all of the assets, including JavaScript files, CSS files, and images.
- Babel: A JavaScript compiler that allows developers to write modern JavaScript code and transpile it to be compatible with older browsers.
- ESLint: A linter that helps to enforce code quality and consistency.
- Jest: A testing framework that provides a simple and easy-to-use API for testing React components and JavaScript code.
- Hot Module Replacement: A feature that allows developers to see the changes they make in real-time without manually refreshing the browser.
Create React App also provides several configuration options, allowing developers to customize their development environment to suit their needs. It supports CSS modules, environment variables, code splitting, and more.
Overall, Create React App is a powerful tool that makes it easy to set up a new React project with minimal configuration, allowing developers to focus on building their applications instead of managing tooling and configuration.
8. react-motion
React Motion is a popular open-source library for building animated user interfaces in React applications. It provides a simple and intuitive API for creating complex animations and interactions, allowing developers to create fluid and engaging user experiences.
React Motion is based on the principles of physics-based animation, which means that a set of parameters such as velocity, acceleration, and damping drives animations. This approach allows for more natural and realistic animations that respond to user input and change over time.
Some of the key features of React Motion include:
- Spring-based animations: React Motion provides a simple and powerful API for creating spring-based animations, which can be used to animate everything from simple transitions to complex interactions.
- Customizable animation parameters: React Motion allows developers to customize the parameters of their animations, including things like spring stiffness, damping, and velocity.
- Performance optimizations: React Motion is designed to be highly performant, with features like batched updates and optimized rendering to minimize the impact on the user interface.
- Easy integration with React: React Motion is designed to work seamlessly with React, allowing developers to build complex animations and interactions using the same familiar API and programming model.
Overall, React Motion is a powerful tool for building animated user interfaces in React applications, allowing developers to create engaging and interactive experiences that respond to user input and change over time.
9. Enzyme
Enzyme is a popular open-source JavaScript testing utility created by Airbnb for testing React components. It provides a set of intuitive and flexible APIs for testing React components’ behaviour and state changes, making it easy to write comprehensive and reliable tests for React applications.
Enzyme offers several features that make it a powerful tool for testing React components, including:
- Shallow rendering: Enzyme provides a shallow rendering API that allows developers to test a component in isolation without rendering its child components.
- Full DOM rendering: Enzyme also provides a full DOM rendering API that allows developers to test a component in the context of its parent and child components.
- Powerful selectors: Enzyme provides a set of powerful selectors that allow developers to find and manipulate components within their tests easily.
- Snapshot testing: Enzyme supports snapshot testing, allowing developers to easily capture the output of a component and compare it against a previously saved snapshot.
- Easy integration with other testing frameworks: Enzyme can be easily integrated with others like Jest, Mocha, and Chai, making it a versatile and flexible tool for testing React applications.
Enzyme is a powerful testing utility that makes writing comprehensive and reliable tests for React components easy. Its intuitive and flexible API, along with its support for shallow rendering, full DOM rendering, and snapshot testing makes it a popular choice among developers for testing React applications.
10. Next.js
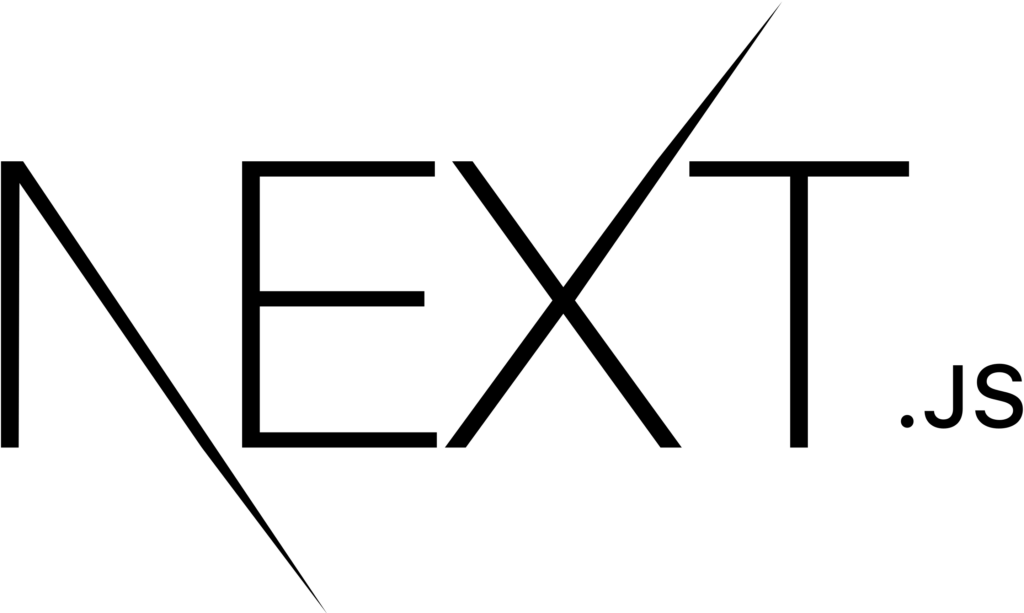
A popular open-source framework for building server-side rendered React applications. It provides features and conventions for building modern web applications, including automatic code splitting, server-side rendering, and static site generation.
Some of the key features of Next.js include:
- Server-side rendering: Next.js provides built-in support for server-side rendering, allowing your application to render on the server and send HTML to the client for improved performance and SEO.
- Automatic code splitting: Next.js automatically splits your code into smaller chunks, allowing your application to load faster and improve performance.
- Static site generation: Next.js allows you to generate a static version of your application, making it easy to deploy to a static hosting provider like Netlify or GitHub Pages.
- File-based routing: Next.js provides a simple and intuitive file-based routing system that makes creating pages and routes in your application easy.
- API routes: Next.js provides a built-in API route system, making creating API endpoints in your application easy.
- CSS-in-JS support: Next.js provides built-in support for popular CSS-in-JS libraries like styled-components and emotion, making creating dynamic and responsive styles in your application easy.
All in all, Next.js is a powerful and versatile framework for building modern web applications. Its built-in support for server-side rendering, automatic code splitting, static site generation, intuitive file-based routing system, and support for popular CSS-in-JS libraries make it a popular choice for building scalable and performant React applications.
Conclusion
This list is not meant to be an exhaustive list of must-know React packages, but React developers need to understand the type of packages available. If this list has helped you, please share the article! Thanks for reading!