In this article, I will attempt to refactor the PlayingCard component that we built in this article. The refactor will convert the component from plain React to MUI. Once we’ve updated the card, we can import this into our Card Game app and use it as the base playing card for each game.
If you’re looking for the final product, you can find it on GitHub here.
Looking at the final PlayingCard component, we can make a few obvious updates.
The first visual change will be the drop shadows that give the card thickness. MUI offers the paper container property that will give a nice paper feel to our card.
Using The Paper Property
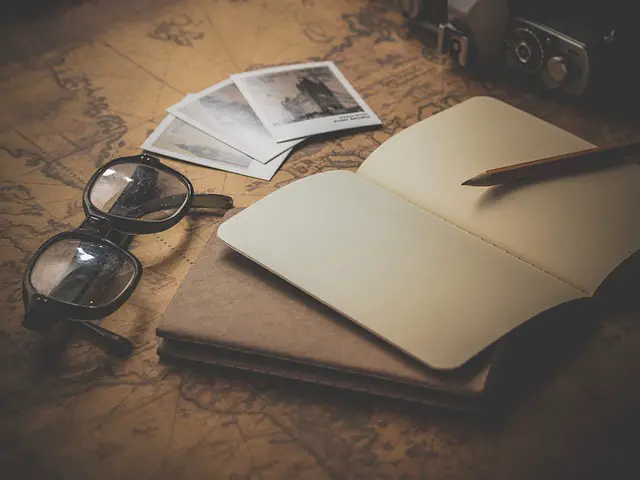
In our PlayingCard component, the card was represented by a div element. Let’s change this to a Paper component. We must replace the opening and ending tags from div to Paper.
Once we’ve updated the tags, we must remove the box shadow style property.
If we were to run the code at this point, you would see the playing card with no shadows looking the same as it did before. This is what we want to see.
Using the elevation attribute, we can recreate the complex box shadow styling we removed earlier. The elevation attribute is an integer (number) from 0-24. 24 is the highest elevation we can give our paper component. Let’s add this to our card now. The Paper component in our code now looks like this:
<Paper
id="theCard"
ref={myRef}
onClick={flipCard}
elevation={24}
style={{
transformStyle: "preserve-3d",
transition: "all 0.5s ease",
transform: "rotateY(180deg)",
width: "240px",
height: "336px",
border: "2px solid transparent",
position: "relative",
backgroundColor: "#F2F2F2",
borderRadius: "10px",
}}
Using Typography In Mui
The next alteration we can make is using Typography instead of standard HTML text elements. We start by making sure we are importing the Roboto font. Following the instructions in the Typography documentation, we need to install the @fontsource/roboto NPM package.
npm install @fontsource/roboto
Once we’ve installed roboto, we update the h1 elements and replace them with Typography components. For example, our top h1 tag becomes:
<Typography top="0px" left="5px" margin="0px" position="absolute" fontSize="50px" color={suitColor} variant="h1" component="h1">
{value}
</Typography>
Updating div Containers to Box
Finally, we can update the div containers in the PlayingCard component to use the MUI Box component instead. After replacing all divs with Boxes, we can update the style attributes to use the MUI sx prop.
And that should do it for now. We’ve taken a plain React component and applied MUI fundamental components. This may not seem like a big deal, but it might become bigger once we start theming our card game site.
Thanks for reading!